How to Show Meta Fields in Frontend
Admin has more flexibility to choose which custom field’s data will show on the front end. This option can be found under “Show data in the post” when editing custom fields under the “Advanced Options” section.
- Add a custom field to the posting form.
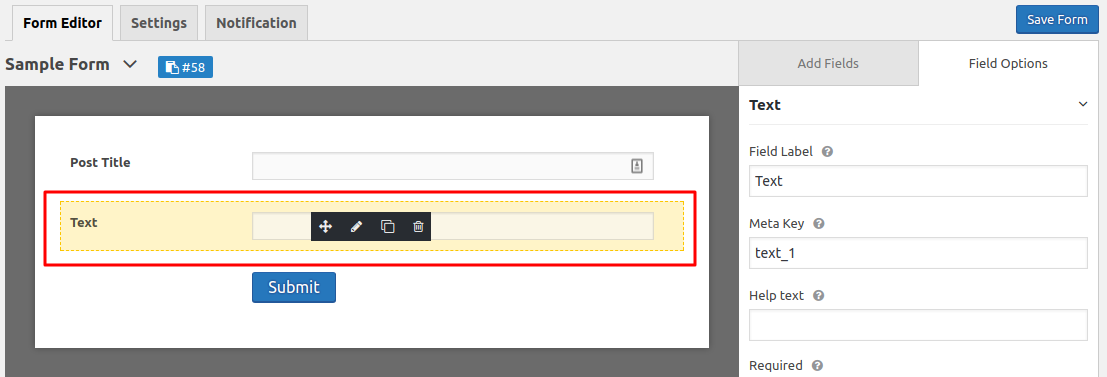
- Edit field options, Under the advanced options section, you will find an option called “Show Data in Post.”
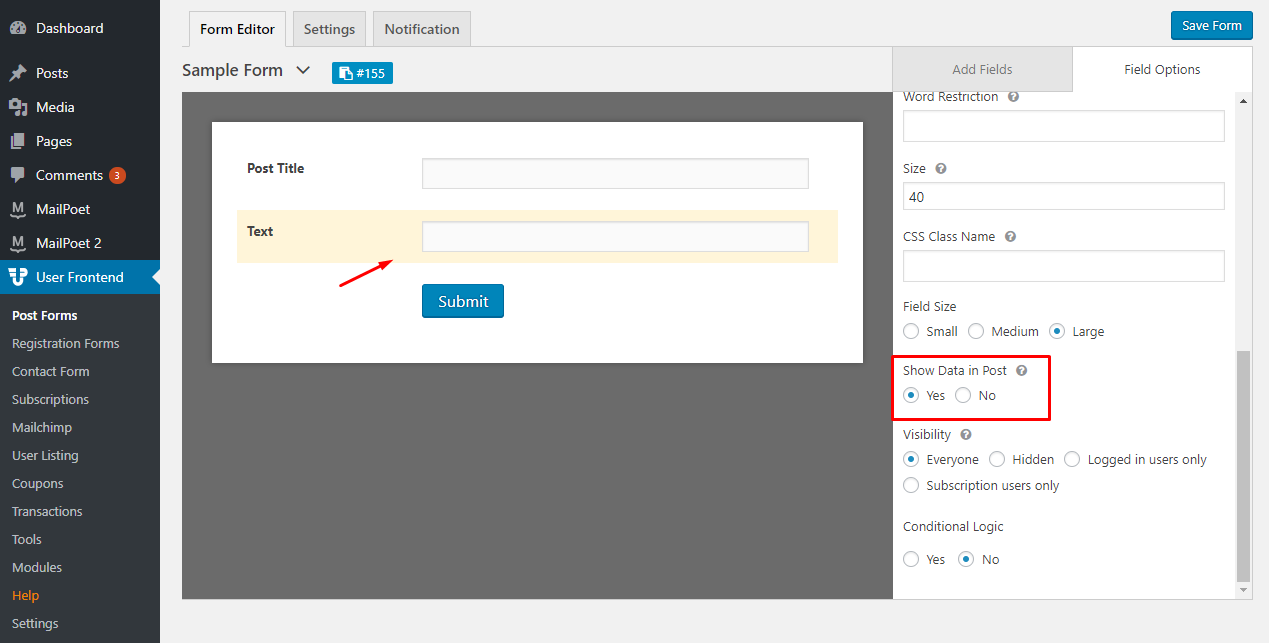
Now, select the option you want and check the post after submitting the form.
Manual Process
Individual Meta
If you want to show individual post meta values in your post, you can use the shortcode[wpuf-meta name=”your_meta_key”]. There are some combinations for this shortcode. You can show your files, images, maps, and multicolumn repeatable fields.
- Simple Fields (text, textarea, select, checkbox, etc.):
[wpuf-meta name="meta_key_name"]
- Google Map:
[wpuf-meta name="meta_key_name" type="map"]
- You can pass other parameters here. Like, height, width and zoom. Example:
[wpuf-meta name="meta_key_name" type="map" height="250" width="450" zoom="12"]
- You can pass other parameters here. Like, height, width and zoom. Example:
- Uploaded Image:
[wpuf-meta name="meta_key_name" type="image"]
- Uploaded File:
[wpuf-meta name="meta_key_name" type="file"]
- Multicolumn Repeat:
[wpuf-meta name="meta_key_name" type="repeat"]
Global Option
There is also a global option to display all the meta fields in your posts. Turning on the “User Frontend->Settings->Frontend Posting->Custom Fields in Post” option will show all your post's custom fields in every post.
Showing fields in the theme
There are situations where you will not find these solutions useful based on your requirements. That's the time when you need to customize your theme. If you want to show the custom fields in your post type, then most of the time, your theme shows the post. If you have a custom post type, then it is responsible for showing that post; if you don't find that file in your theme, then it is handling that job. If you want to display a custom field, then this code will show that. You can show text, textarea, dropdown, date, radio, etc.
<?php
global $post;
$address_fields = get_post_meta( $post->ID, 'address_field', true );
foreach ( $address_fields as $field ) {
echo $field . "<br>";
}
?>
Image or File: If you have a image or file type custom field, then you can show them like this code below. Here we are showing the image thumbnail and linking the image to the full size image.
$images = get_post_meta( $post->ID, 'mey_key_name');
if ( $images ) {
foreach ( $images as $attachment_id ) {
$thumb = wp_get_attachment_image( $attachment_id, 'thumbnail' );
$full_size = wp_get_attachment_url( $attachment_id );
printf( '<a href="%s">%s</a>', $full_size, $thumb );
}
}
Google Map: If you have a custom field named location
, you can show the map with this code:
<?php
echo wpuf_shortcode_map( 'location', $post->ID );
?>
Single Column Repeat: If you have a single column repeatable field with meta key repeat
, we can show them in an unordered list like this:
$repeat_field = get_post_meta( $post->ID, 'repeat', true );
if ( $repeat_field ) {
$values = explode( '| ', $repeat_field );
echo '<ul>';
foreach ($values as $value) {
echo "<li>Value: $value</li>";
}
echo '</ul>';
}
Multi-Column Repeat: If you have a multi-column repeatable field with a meta key multi_repeat
and columns Name and Email, we can show them in an unordered list like this:
$repeat_field = get_post_meta( $post->ID, 'multi_repeat' );
if ( $repeat_field ) {
echo '<ul>';
foreach ($repeat_field as $field) {
$values = explode( '| ', $field );
echo "<li>Name: {$values[0]}, E-Mail: {$values[1]}</li>";
}
echo '</ul>';
}